Get started
Coassemble Headless provides a way to embed rich learning content that you have created in Coassemble in your own platform. It can also optionally provide a white-labelled course creation tool right in your own platform. For a more detailed explanation about Coassemble Headless and it's potential use cases, please see this page
The following is guide on how to setup a basic implementation of Coassemble Headless in a few simple steps.
1. Create courses
Begin by creating courses in Coassemble. We will fetch these courses in an upcoming step to display them in your platform.
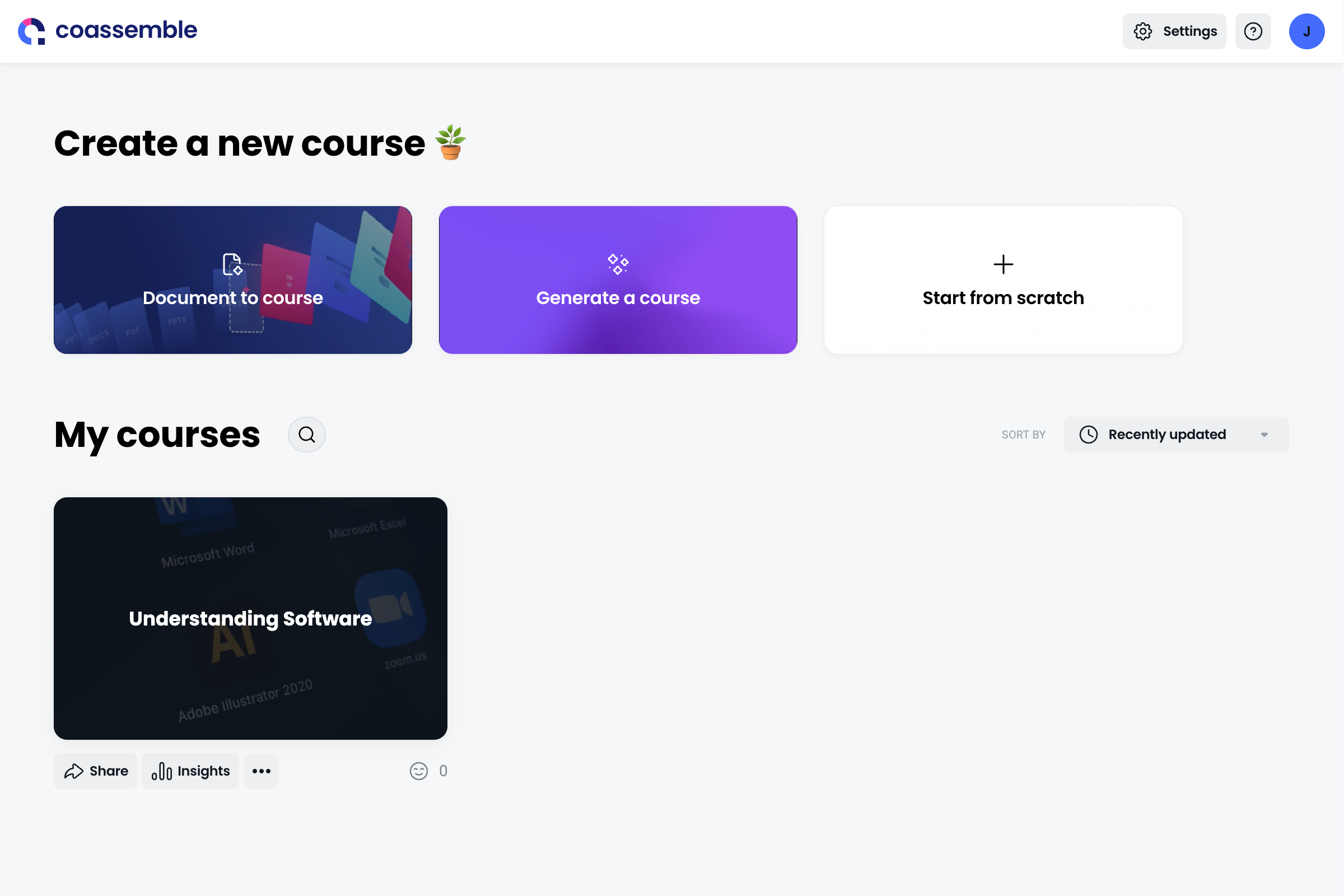
2. Get your API credentials
If you haven't already, enable Headless in your workspace by navigating to the Add-ons section of the settings menu and clicking on Headless. You will initially be in 'test mode' allowing you to configure your implementation before purchasing and going live.
Once you have completed the entry survey and enabled Headless, you will be shown your API credentials including your user ID and API key. Store these securely as we will not show them again.
If you lose your API credentials, you can regenerate them at any time from this settings menu.
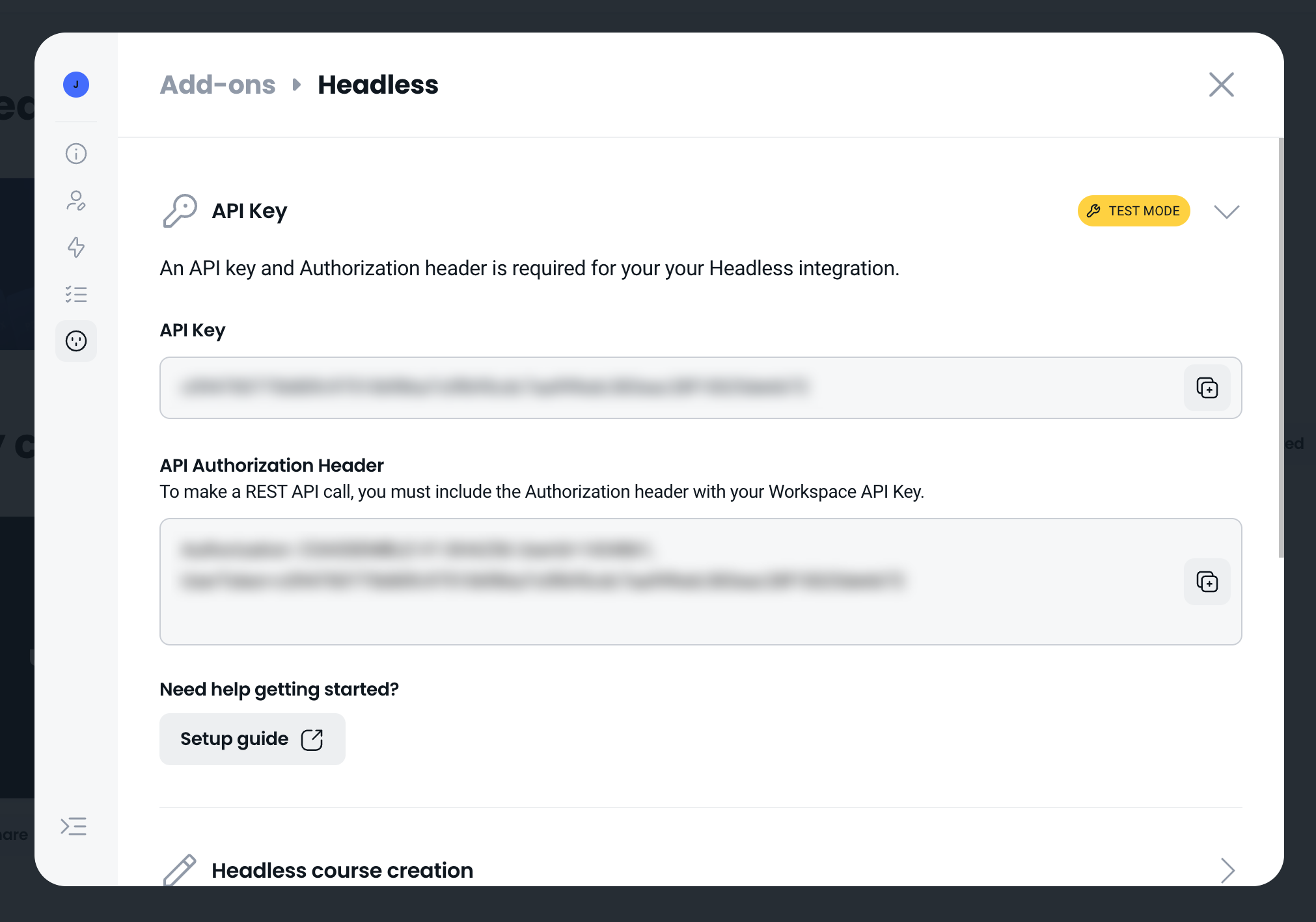
3. Fetch your courses via the API
Using the API credentials you obtained in the previous step, you can now fetch your courses via the API.
For full details on this endpoint, please see the API reference documentation.
Request example
const response = await fetch(
'https://api.coassemble.com/api/v1/headless/courses',
{
method: 'GET',
headers: {
'Authorization': 'COASSEMBLE-V1-SHA256 UserId={USER_ID}, UserToken={API_KEY}'
}
}
);
const courses = await response.json();
Response example (truncated)
[
{
"id": 1234,
"title": "Understanding Software",
"description": null,
"image": "...",
"thumbnail": "...",
"active": true,
"identified": false,
"private": false,
"key": "YOURCOURSEKEY",
"source": null,
"emails": []
}
]
4. Request a signed URL to embed your course
Now that you have your courses, you can request a signed URL to embed one of them in your platform.
Note that you must also pass an identifier
parameter. It is recommended that you use a unique identifier from within your system such as a user ID.
For full details on this endpoint, please see the API reference documentation.
Request example
const response = await fetch(
'https://api.coassemble.com/api/v1/headless/course/view?id={COURSE_ID}&identifier={IDENTIFIER}',
{
method: 'GET',
headers: {
'Authorization': 'COASSEMBLE-V1-SHA256 UserId={USER_ID}, UserToken={API_KEY}'
}
}
);
const url = await response.json();
Response example
"https://coassemble.com/embed/{TOKEN}"
5. Embed the course URL in your platform
Finally, you can embed the signed URL you received in the previous step in your platform.
🎉 You're now ready to embed Coassemble courses in your platform!
To refine your implementation, see the API reference documentation for more advanced features and use cases.
Embedding a course
6. Handle progress events (optional)
When embedding courses for viewing as a learner, you can optionally handle progress events. This allows you to track the progress of your users through the content, and respond in your application accordingly.
For a complete list of available events and their descriptions, see our Events documentation.
Listening for progress events
window.addEventListener('message', message => {
if (message.origin !== 'https://coassemble.com') return;
const payload = JSON.parse(message.data);
if (data.type === 'course') {
if (data.event === 'progress') {
// Handle progress event
}
if (data.event === 'complete') {
// Handle complete event
}
}
});
Progress event example
{
"type": "course", // [course, screen]
"event": "complete", // [start, end, progress, complete]
"data": {
"id": 123, // Object ID
"progress": 100, // Progress percentage as an integer
"completed": true // Whether the object is completed
}
}
7. Request a signed URL to embed our course creation tool (optional)
If you would like to allow your users to create courses in your platform, you can request a signed URL to embed our course creation tool. Note that you must have Headless course creation enabled in your workspace to use this feature.
The identifier
parameter is also required for the edit
action. It is recommended that you use the same unique identifier from within your system such as a user ID. You can optionally pass this identifier
as a parameter when fetching courses to fetch only courses created by that user.
The id
parameter is optional. If provided, the course builder will open with the course specified. If not provided, the course builder will open with a new course.
The colorPrimary
parameter is optional. If provided, the course builder will open themed with the specified color.
You may also pass a flow
parameter to specify the flow of the course builder.
Flow | Description |
---|---|
ai | Open course builder with the AI flow. This flow will guide the user through creating a course using our AI-powered course creation tool. |
document | Open course builder with the document flow. This flow will guide the user through creating a course by converting a document. |
preview | Open course builder in preview-only mode. This flow will allow the user to preview a course without being able to edit it. |
Similar to embedding a learner course, you may also optionally listen for events:
For a complete list of available events and their descriptions, see our Events documentation.
For full details on this endpoint, please see the API reference documentation.
Request example
const response = await fetch(
'https://api.coassemble.com/api/v1/headless/course/edit?identifier={IDENTIFIER}flow=document',
{
method: 'GET',
headers: {
'Authorization': 'COASSEMBLE-V1-SHA256 UserId={USER_ID}, UserToken={API_KEY}'
}
}
);
const data = await response.json();
Response example
"https://coassemble.com/embed/{TOKEN}"
Listening for events
window.addEventListener('message', message => {
if (message.origin !== 'https://coassemble.com') return;
const payload = JSON.parse(message.data);
if (data.type === 'back') {
// Close the builder in your application
}
});